enum and typedef
To make the coding of a program more intelligible, it is sometimes useful to replace integer values such as 1, 2, 3 etc. with meaningful names. An enumeration is created with the enum keyword, and is an ordered collection of names that represent integer values. Unless otherwise specified, the first name in the collection represents 0, the second name represents 1, and so on. In the statement below, for example, the names false and true become synonymous with the integer values 0 and 1.
enum {false, true};
It is perfectly possible to define an enumerated data type using the typedef keyword. This keyword allows you to give a new name to an existing data type - you can think of it as an alias - with the aim of making the source code easier to follow. This might be useful, for example, if you wanted to create a Boolean data type for your program. A variable of type Boolean can take one of two values - false or true (represented by the values 0 and 1 respectively). While many programming languages have a specific Boolean data type, C does not. We could therefore create a Boolean data type with the following statement:
typedef enum {false, true} boolean;
We can now create a variable of this type (boolean) using a statement such as the following:
boolean error = false;
Supposing you want to be able to handle a situation in which the user enters a value that does not match one of the expected responses. Your program should be able to "trap" such an error, and report its occurrence to the user. The short program below expects the user to enter a temperature value, and then respond to a question about whether or not it is raining by pressing either "Y" or "N" (for "yes" or "no"). If the user responds to this question with anything other than "Y" or "N", the variable input_error (of type boolean) is set to true (1), indicating that an input error has occurred. The variable is initialised to false (0), so if a valid response is given, the error message will not be displayed.
#include <stdio.h>
#include <string.h>
void main()
{
typedef enum{false, true} boolean;
const char yes='Y';
const char no='N';
char response;
char item[25];
int temp=0;
boolean input_error=false;
printf("What is the temperature outside today? ");
scanf("%d", &temp);
fflush(stdin);
printf("Is it raining outside? Answer Y (yes) or N (no) ");
scanf("%c", &response);
fflush(stdin);
if(response==yes)
if(temp < 60)
strcpy(item, "raincoat and umbrella");
else
strcpy(item, "umbrella");
else
if(response==no)
if(temp < 60)
strcpy(item, "overcoat");
else
strcpy(item, "jacket");
else
input_error = true;
if(input_error)
printf("INPUT ERROR - your response must be Y or N.\n");
else
printf("Before you leave the house take your %s.\n", item);
getchar();
}
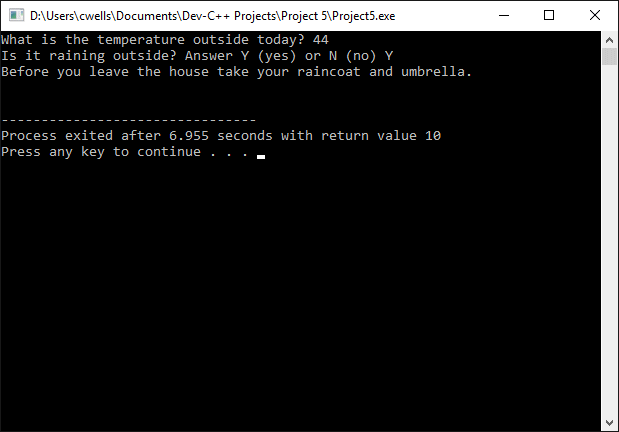
The program output for valid input
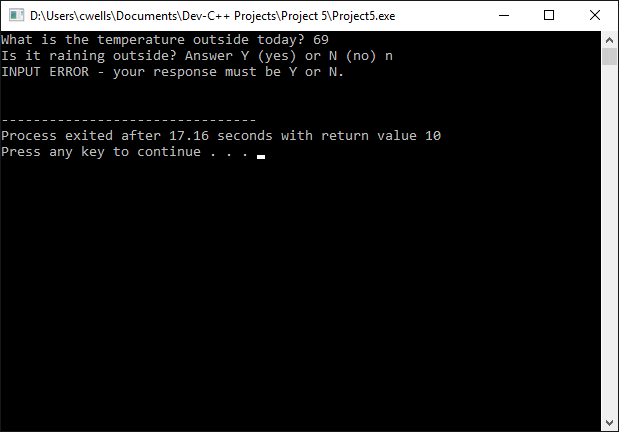
The program output if an input error has occurred
Obviously, the program itself has a few shortcomings. If you input a non-numerical value when asked for the temperature, the temperature variable will have the default value of zero, because we have not included any code to trap this kind of imput error. The program will also only accept the upper case characters 'Y' and 'N', and will report an error if we enter 'y' or 'n' in response to the question "Is it raining outside?". Nevertheless, it serves to demonstrate the creation and use of an enumerated datatype (boolean).