Basic Input and Output
C++ provides a number of standard library functions to enable users to interact with an application by, for example, displaying information on the screen, or entering data via the keyboard. C++ uses streams to perform many text input and output operations. The term stream refers to the sequential flow of data to a standard output object (normally the display monitor) or from a standard input object (normally the keyboard). The C++ library header file iostream contains declarations for the standard input and output stream objects.
Standard output
By default, a C++ program's text output goes to the display monitor, and the stream object defined for this purpose is cout. The cout object is used together with the insertion operator (<<), as shown below.
cout << "Here is some text"; // outputs some text
cout << 120; // outputs a number
In the examples given above, the insertion operator (<<) inserts data into the cout stream. The insertion operator can be used more than once in a statement to send different types of date to the cout stream at the same time, as shown below.
cout << "Here is a number: " << 247;
Note that successive cout statements do not appear on separate lines unless we explicitly include a new-line escape character (\n) at the point where a new line is required. The following code fragment demonstrates the use of the new-line escape sequence:
cout << "This is line one.\n";
cout << "This is line two.\nThis is line three";
This produces the following output:
This is line one.
This is line two.
This is line three.
Standard input
By default, the standard input device in C++ is the keyboard, and the stream object defined for this purpose is cin. The cin object is used together with the extraction operator (>>). An example program is given below.
// Example Program 1
#include <iostream>
using namespace std;
int main ()
{
string name, s;
int age;
cout << "Enter your name: "; // get user name
cin >> name;
cout << "\n\nEnter your age: "; // get user age
cin >> age;
cout << "\n\nThank you, " << name << " aged " << age;
cout << "\n\n\nPress ENTER to continue.";
getline( cin, s );
getline( cin, s );
return 0;
}
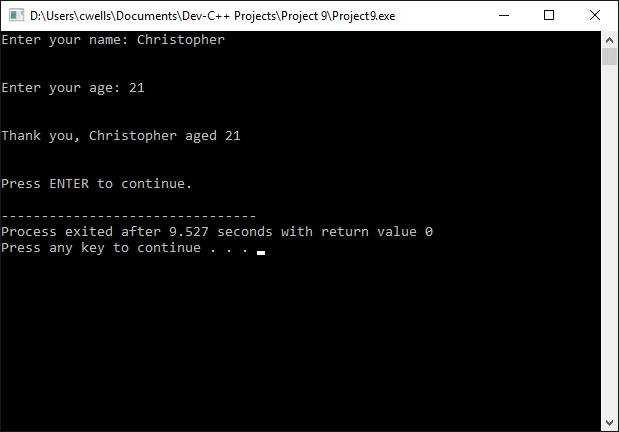
The output from example program 1
The cin object can only process keyboard input after the ENTER key has been pressed.
The getline() function
While cin is useful, input is limited to strings that do not contain any white space. If we need to input a string that contains spaces or other white space characters, we can use the getline() function. We have already used this function in previous examples to wait for the user to press the ENTER key. The following short program demonstrates the use of the getline() function.
// Example Program 2
#include <iostream>
using namespace std;
int main ()
{
string s;
cout << "Please enter your full name: ";
getline( cin, s ); // get user's full name
cout << "\n\nThank you, " << s;
cout << "\n\n\nPress ENTER to continue.";
getline( cin, s );
return 0;
}
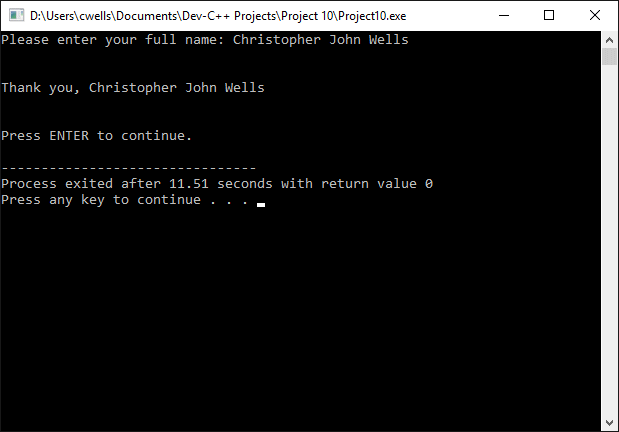
The output from example program 2
Notice that we have called the getline() function twice using the same string identifier - once to obtain the user's full name, and once to capture a single user keystroke (ENTER). The value initially stored in the variable s (the user's full name) is overwritten by the second call to getline().
The stringstream class
The stringstream class is defined in the library header file sstream, and provides an interface that allows strings to be manipulated as if they were input or output streams. This enables us to use the insertion and extraction operators with string variables. The following code fragment extracts an integer from a string:
string numStr = "123";
int numInt;
stringstream(numStr) >> numInt;
The code example above declares a string variable and initilises it to "123". The stringstream class's constructor method (more about these later) is called in order to create an object of the type stringstream using the contents of the string variable numStr. The extraction operator can then be used to extract the integer value represented, and store the value in the integer variable numInt. The following short sample program further demonstrates the use of sstream.
// Example Program 3
#include <iostream>
#include <sstream>
using namespace std;
int main ()
{
string s;
float price = 0;
int qty = 0;
cout << "Please enter a price: ";
getline( cin, s );
stringstream(s) >> price;
cout << "\n\nPlease enter a quantity: ";
getline( cin, s );
stringstream(s) >> qty;
cout << "\n\nTotal Cost: " << price * qty;
cout << "\n\n\nPress ENTER to continue.";
getline( cin, s );
return 0;
}
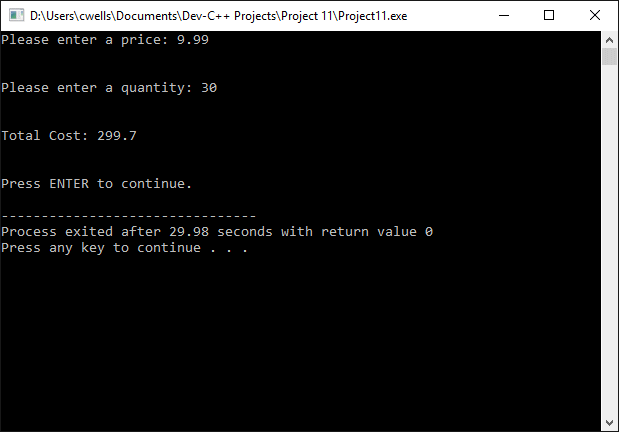
The output from example program 3
In this program, we do not get numeric values from the standard input stream directly. Instead, we use the input to create an object of type stringstream, and extract the numeric value from that. The advantage of doing this is that we can exercise more control over the way in which numerical data is input to our application, since we have separated the process of obtaining the input from that of interpreting it. This method is thus the preferred one in applications that involve a high volume of numerical input.