Beyond "Hello World!" with Visual Basic
We are now going to create a more useful Visual Basic application - an image viewer application that lets you view graphic image files on your computer. Start Visual Studio 2022, and create a new project (select Windows Forms Application, as you did for the "Hello World!" program). Name the project "ImageViewer". Once the project has been created, you should see a screen like the one shown below.
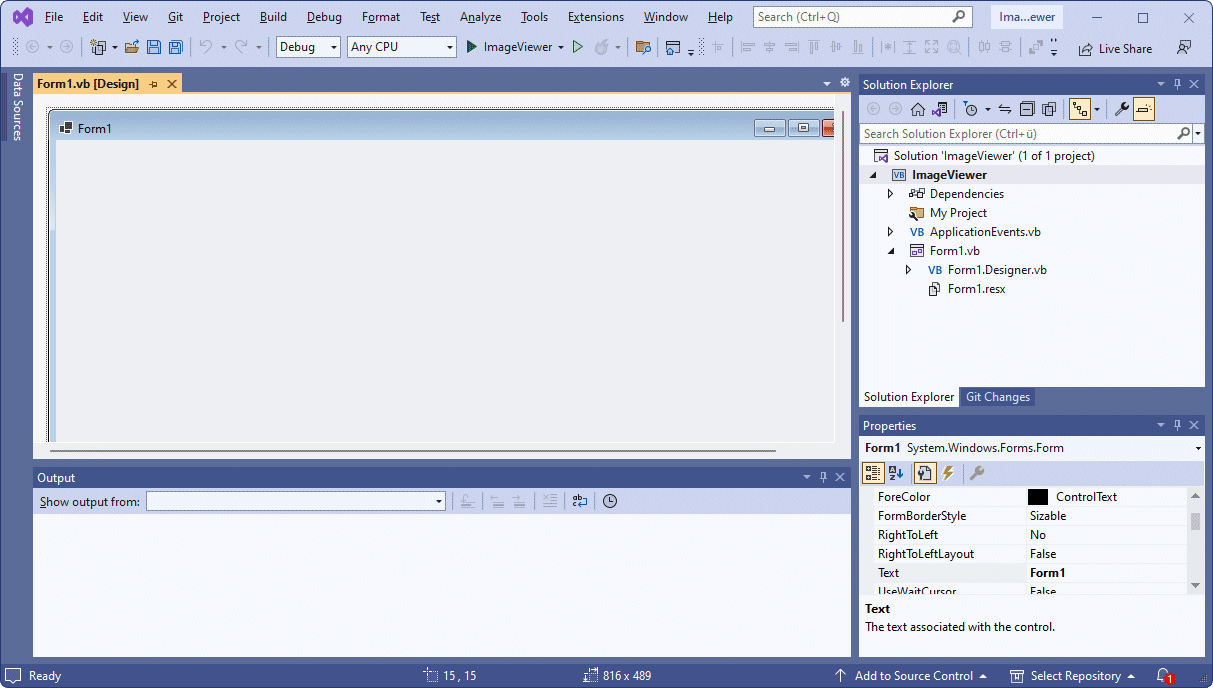
The ImageViewer project
- Click anywhere in the default form. Its properties should be displayed in the Properties window. Note that by default the form will be called by the generic name Form1.
- Scroll to the top of the properties list until you see the (Name) property.
- Click the (Name) property, and change the text from Form1 to ViewForm.
- Right-click the Form1.vb object in the Solution Explorer window.
- Choose Rename from the context menu that appears.
- Change the name from Form1.vb to ViewForm.vb (note for future reference that if you change the form's filename first, it will be renamed automatically)
- Click on the form once again to display its properties and scroll to the Text property.
- Change the text to "Image Viewer".
- In the Solution Explorer window, right click on the project name ("Image Viewer") and select Properties from the drop-down menu. The application's Properties window will appear. Scroll down to the Startup Object property, and use the drop-down menu to select ImageViewer.ViewForm.
Note that, by default, the project's Startup Object property will be set to ImageViewer.Form1. In previous versions of Visual Studio, changing the name of Form1.vb would automatically update the Startup Object property, but this no longer seems to be the case. The above step is therefore very important, otherwise the application will not compile correctly.
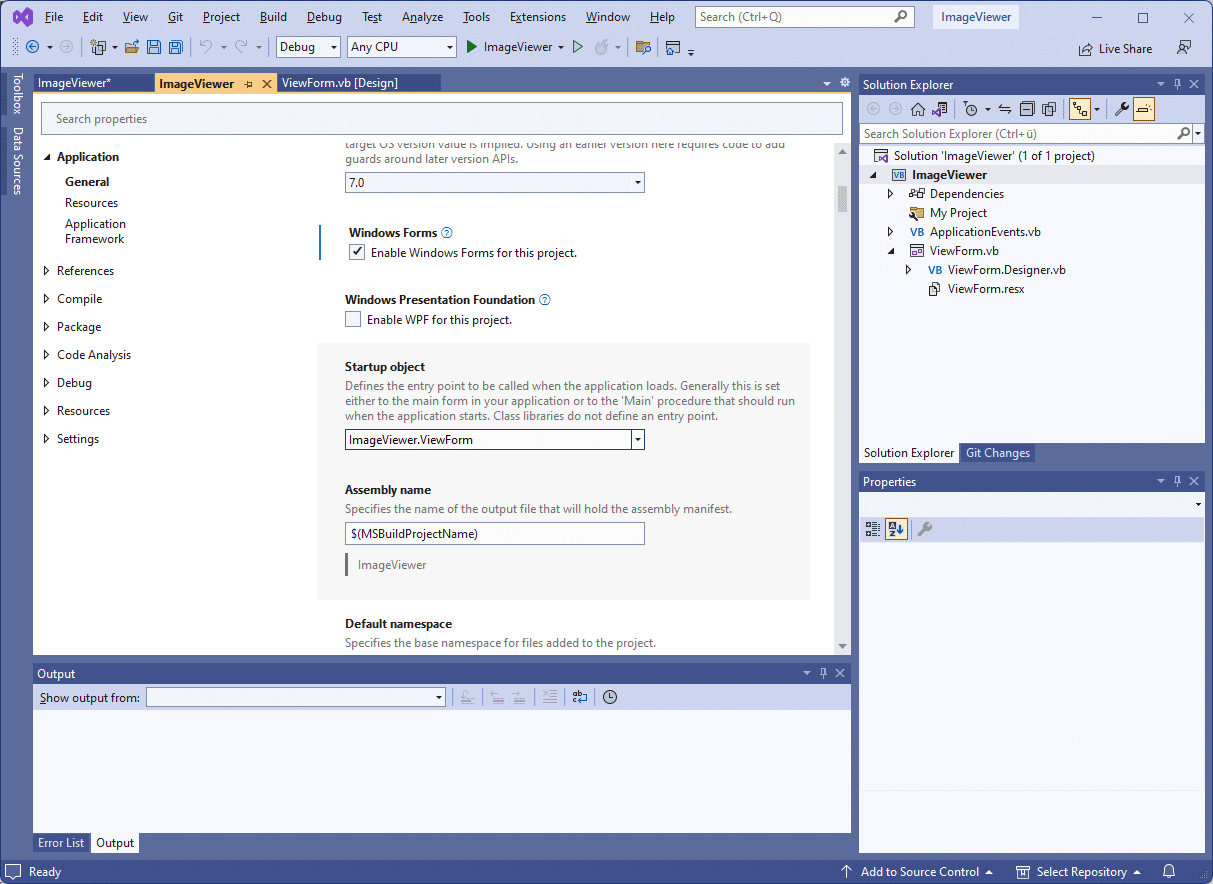
The ImageViewer project's Properties window is displayed
- Click on the Save All button in the toolbar at the top of the screen (that's the icon that looks like two floppy disks).
Visual Basic will create the necessary folders for your project, using the name you gave the project as you created it, and save the project files to the default location (to change the default location for your project folders, select Options > Projects and Solutions > Locations from the Tools menu).
By default, Visual Basic will create a solution directory in which the project directory will reside. Both the solution directory and the project directory will have the same name, i.e. the name you chose for your project (in this case, "ImageViewer").
It might be a good idea at this point to make a note of the location of the directory to which your project will be saved (right-click on MyProject > Open Folder in File Explorer in the Solution Explorer window to open the directory and see your project files).
- Download the icon for the application here, unzip it, and save it to your project folder.
- Select the ImageViewer form once more. In the Properties window, scroll to the Icon property and click on it once to select it.
- A button with three dots will appear to the right of the Icon property. Click on the button once.
- The Open dialogue box that appears. Browse to the ImageViewer project folder, click on the file ImageViewer.ico to select it, and then click on the Open button. The icon should now appear in both the form itself, and in the Icon property field.
- In the Properties window for the form, find the Size property and change the values to 400 and 325 (these values represent the width and height in pixels, respectively). The Properties window should now look like the illustration below (note that you can also resize a form by dragging its borders with the mouse, as well as programmatically).

- Save your project once more using the Save All button.
Our application form is now exactly how we want it, and we can create the user interface by adding some controls to it. At some point, we will need to write some program code to make things happen when we use the application, but for now we will concentrate on creating the user interface.
- Click the Toolbox tab (on the left hand side of the screen) to display the toolbox window, and click the plus sign next to Common Controls to see the most commonly used controls. If the Toolbox tab is not visible, you can open it from the View menu (View ► Toolbox).
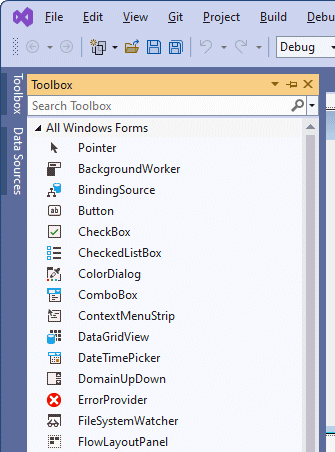
- Double-click on the Button item in the toolbox. Visual Basic creates a new button and puts it in the upper-left corner of the form.
- Set the property values for the button as shown in the table below.
Property | Value |
---|---|
Name | btnSelectImage |
Location | 295, 10 |
Size | 85,23 |
Text | Select Image |
- Right-click the Select Image button and choose Copy from the context menu that appears.
- Right-click anywhere on the form and choose Paste from the form's shortcut menu. The new button will appear centred on the form, and is selected by default. It will be identical to the first button, apart from its name and position. Change its properties as shown in the table below.
Property | Value |
---|---|
Name | btnExit |
Location | 295, 40 |
Text | Exit |
- Double-click on the PictureBox item in the toolbox to add a picture box control to the form, and set its properties as shown in the table below.
Property | Value |
---|---|
Name | picShowImage |
BorderStyle | FixedSingle |
Location | 8, 8 |
Size | 282, 275 |
The form should now look like the one shown below.
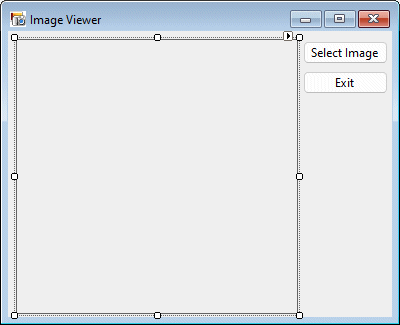
- Click on the the Save All button again to save your work.
- In the toolbox, expand the Dialogs category, and double-click on the OpenFileDialog item to add it to the form. This control will not be visible to the user in the normal course of events, and will therefore appear at the bottom of the design window, underneath the form, as shown below.
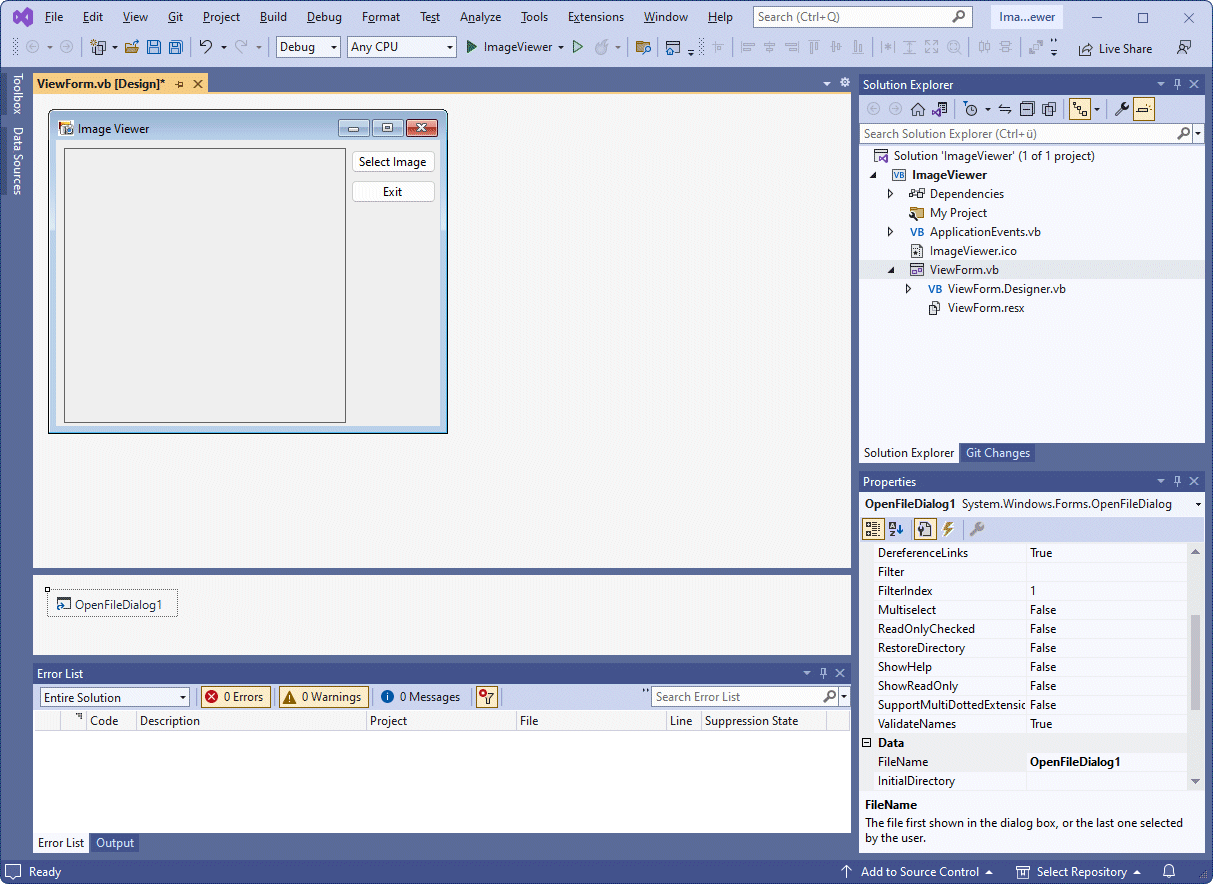
- Make sure the OpenFileDialog control is selected, and set its properties to those shown below.
Property | Value |
---|---|
Name | ofdSelectImage |
FileName | (this should be blank) |
Filter | Windows Bitmaps|*.BMP|JPEG Files|*.JPG |
Title | Select Image |
The filter property determines the type of files that will be displayed in the Open File dialogue box when the application runs. The text in front of the pipe symbol ("|") is the descriptive name given to the file type, while the text that follows it is the pattern used to filter files. A pipe symbol is also used to separate filters.
Some program code is now needed to make the program actually do something when a user interacts with it. Program code is executed in response to some event, such as the program being started, or a user clicking on a button. We will need to add some code that allows the user to browse their hard drive for an image file and display it, and we will also need some code to close the program when the user clicks on the Exit button.
- Double-click the Select Image button now to access its Click event in the code window. Visual Basic creates an event handler template for the button's default event (a user click). The cursor will be positioned at the point where you need to insert code.

- Enter the following code at the cursor:
If ofdSelectImage.ShowDialog = DialogResult.OK Then
picShowImage.Image = Image.FromFile (ofdSelectImage.filename)
Me.Text = "Picture Viewer (" & ofdSelectImage.FileName & ") "
End If
Your code should now look like the illustration below.
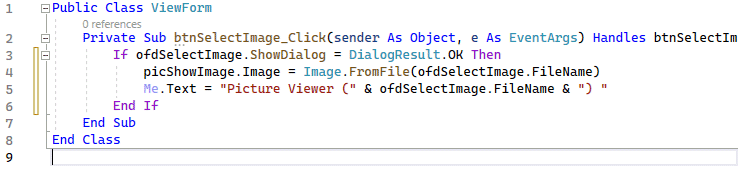
The first line of code you entered triggers the ShowDialog() method of the OpenFileDialog control that tells it to show the Open dialogue box that allows the user to browse their computer's hard drive for an image file. The method returns a value that indicates success or failure, which is then compared to a predefined result (DialogResult.OK).
If the user selects a file, the code between the If ... End If statements is executed (note that Visual Basic will automatically add the End If, as well as capitalising your code to match control names if necessary, and taking care of indentation).
The second line of code you entered actually displays the image stored in the selected file in the picture box, while the third line of code displays the path and filename of the image in the application's title bar. Now we just need some code to make the Exit button work.
- If the form is not currently visible, click on the tab at the top of the code window that says ViewForm.vb [Design] to switch to the form designer.
- Double-click the Exit button, and type the line of code shown below in the button's Click event handler. This statement closes the form, and since this is the only form in the application, it closes the application as well.
Me.Close()
- Your code should now look like the illustration below. Click on the Save All button, then run the program by pressing the F5 key (or the Start button on the toolbar).
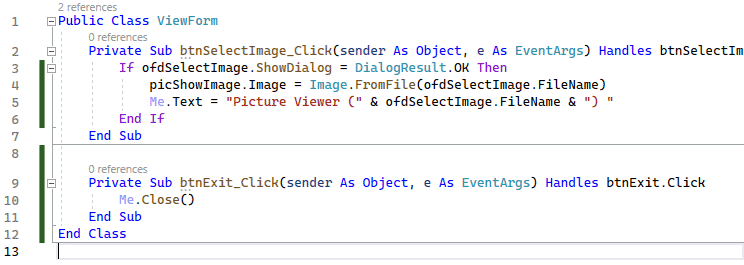
- When the application opens, click on the Select Image button to display the Select Image dialog box (see below), and find a suitable image to display.
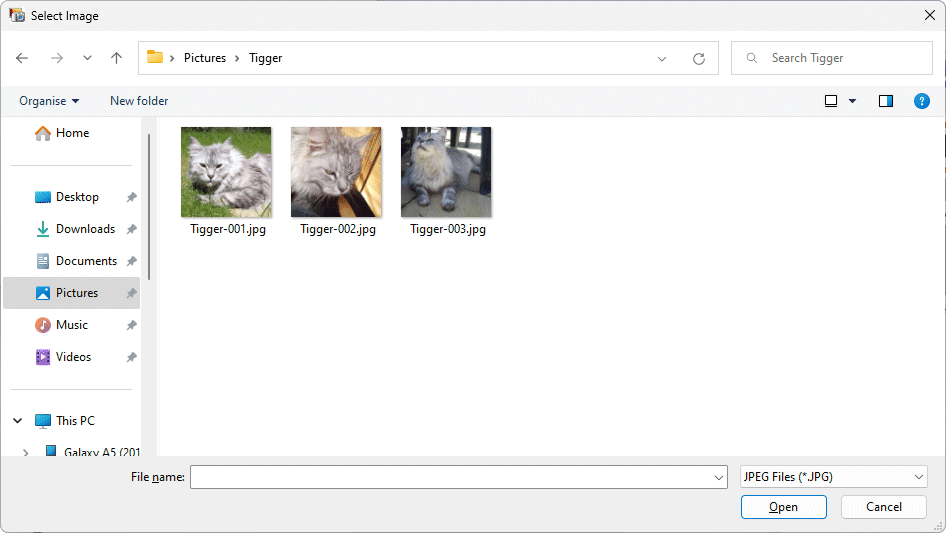
- Once you have found an image and selected it, either double-click on it, or click on the Open button do display the image.

Of course, if the image is very small, it will not fill the display area. If it is very large, only the top left-hand portion of the image will be visible. We deliberately chose pictures here that would fit into the space provided. We could refine the program in due course to allow images to be scaled to fit the display area.
It is also a relatively easy matter to modify the application to display images in other file formats (the PictureBox control can also display image files with the extensions .ico, .emf, .wmf and .gif). You can amend the Filter property of the OpenFileDialog control to include any of these file types if required.