Introduction to Visual Basic Classes and Objects
Visual Basic is an object-oriented programming language. An object encapsulates both data and the methods (procedures) that act on the data. The details of the data structures used and the implementation of the methods are hidden within the object. All the programmer needs to know in order to use an object is what tasks the object can perform, and the parameters required by the object in order to perform those tasks.
When you click on the Button item in the Visual Basic toolbox, a button object is placed on your form. Click on the Button item again, and another button object that looks exactly like the first button object will be placed on your form. Each of these objects has the same properties and methods, with the same default value for each property except the (Name) property. In fact, clicking on any of the items in the Visual Basic toolbox will result in a predefined object of one kind or another being placed on your form.
The button objects on your form are all instances of a class. Essentially, a class is a template from which an object is created, and specifies the properties and methods that will be common to all objects of that class. Each text box you place on a form is therefore an instance of the class TextBox. Once you have created the text box object, you can set its properties and invoke its methods. For example, you can set the Text property by assigning it the value of a string variable. Or you could clear the contents of the text box by calling the Clear() method.
As programmers, we don't need to know how the methods provided by Visual Basic's built in objects are implemented. We just need to know what properties we can access, and what methods are available to us. This changes when we start creating our own user-defined classes; we need to to know a little more about how classes work. With that in mind, we are going to create an application that calls on the services of a user-defined class.
- Open a new project called "Classes" and create a form that looks like the one illustrated below.
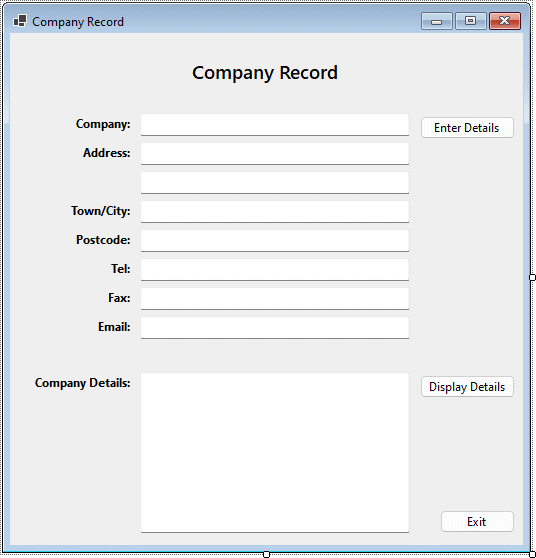
The Classes program interface
- Rename the form's controls as shown in the table below.
Control | Name |
---|---|
Form | frmClasses |
TextBox 1 | txtCompany |
TextBox 2 | txtAddress01 |
TextBox 3 | txtAddress02 |
TextBox 4 | txtTown |
TextBox 5 | txtPostCode |
TextBox 6 | txtTel |
TextBox 7 | txtFax |
TextBox 8 | txtEmail |
TextBox 9 | txtDetails |
Button 1 | cmdEnter |
Button 2 | cmdDisplay |
Button 3 | cmdExit |
Creating the CCustomer class
It is a convention to precede the name of a class with the letter 'C'. We will therefore use the name CCustomer for our class. Use the following steps to create the CCustomer class:
- From the Project menu, select Add Class...
- Change the default file name to "CCustomer.vb" and click on Add
- The code editor window for the class will open with the following code already created for you:
Public Class CCustomer
End Class
Applications communicate with objects through the properties and methods they expose. When you program with Visual Basic, you are working with objects (like the controls you place on a form) by accessing their properties and invoking their methods. One of the first things we should perhaps look at is the issue of encapsulation - a central theme in object-oriented programming. Encapsulation essentially means hiding both the complexity of an object and the object's data.
Hiding complexity essentially means that the only thing a user of the object has to worry about is what the object does - not how it does it. The object offers methods for carrying out various tasks. How those methods are implemented internally is of no concern to the user. In principle, as long as each method's inputs and outputs remain the same, the code that implements the functionality of an object's methods can be completely rewritten (perhaps in order to make the object's implementation more efficient) without affecting the way in which the object is used.
Hiding data essentially means hiding an object's member variables from view within the object by declaring them as private using the Private keyword. Private member variables are only accessible using the object's publicly accessible methods. Although it is perfectly possible to make an object's member variables publicly accessible, it is considered to be poor programming practice for a number of reasons. For one thing, it means that an object's variables can potentially be accessed and modified from anywhere in the program - which becomes a problem when you suddenly find the variables taking on unexpected values and have to track down the culprit.
Our CCustomer class has a number of member variables, which we are going to declare as private for the reasons discussed above, and because there is no compelling reason not to do so. In order to be able to access our member variables, we will provide two methods for each variable - one to set the variable's value, and another to retrieve it. Such methods are often referred to as accessor methods.
- Add the following code (we have been quite liberal with comments!) to the class definition:
'These are the member variables used to hold data.
'The word "Private" means they cannot be accessed directly.
Private mCompany, mAddress01, mAddress02, mTown As String
Private mPostCode, mTel, mFax, mEmail As String
'This procedure retrieves the value of the variable mCopmpany
Public Function GetCompany() As String
GetCompany = mCompany
End Function
'This procedure assigns a value to the variable mCopmpany
Public Sub SetCompany (strValue As String)
mCompany = strValue
End Sub
'This procedure retrieves the value of the variable mAddress01
Public Function GetAddress01() As String
GetAddress01 = mAddress01
End Function
'This procedure assigns a value to the variable mAddress01
Public Sub SetAddress01 (strValue As String)
mAddress01 = strValue
End Sub
'This procedure retrieves the value of the variable mAddress02
Public Function GetAddress02() As String
GetAddress02 = mAddress02
End Function
'This procedure assigns a value to the variable mAddress02
Public Sub SetAddress02 (strValue As String)
mAddress02 = strValue
End Sub
'This procedure retrieves the value of the variable mTown
Public Function GetTown() As String
GetTown = mTown
End Function
'This procedure assigns a value to the variable mTown
Public Sub SetTown (strValue As String)
mTown = strValue
End Sub
'This procedure retrieves the value of the variable mPostCode
Public Function GetPostCode() As String
GetPostCode = mPostCode
End Function
'This procedure assigns a value to the variable mPostCode
Public Sub SetPostCode (strValue As String)
mPostCode = strValue
End Sub
'This procedure retrieves the value of the variable mTel
Public Function GetTel() As String
GetTel = mTel
End Function
'This procedure assigns a value to the variable mTel
Public Sub SetTel (strValue As String)
mTel = strValue
End Sub
'This procedure retrieves the value of the variable mFax
Public Function GetFax() As String
GetFax = mFax
End Function
'This procedure assigns a value to the variable mFax
Public Sub SetFax (strValue As String)
mFax = strValue
End Sub
'This procedure retrieves the value of the variable mEmail
Public Function GetEmail() As String
GetEmail = mEmail
End Function
'This procedure assigns a value to the variable mEmail
Public Sub SetEmail (strValue As String)
mEmail = strValue
End Sub
Using the CCustomer class
An object is an instance of a class. We are going to add some code to our application's form that creates an instance of the CCustomer class, which is defined in the module CCustomer.vb. Our program will communicate with this class object via it's interface. The interface consists of the methods and properties defined within the class declaration. Every instance of the class will have these methods and properties.
- Add the following code to the form's class definition:
Dim strDetails As String
Dim customer As New CCustomer
Private Sub cmdExit_Click(sender As Object, e As EventArgs) _
Handles cmdExit.Click
End
End Sub
Private Sub cmdEnter_Click(sender As Object, e As EventArgs) _
Handles cmdEnter.Click
customer.SetCompany(txtCompany.Text)
customer.SetAddress01(txtAddress01.Text)
customer.SetAddress02(txtAddress02.Text)
customer.SetTown(txtTown.Text)
customer.SetPostCode(txtPostCode.Text)
customer.SetTel(txtTel.Text)
customer.SetFax(txtFax.Text)
customer.SetEmail(txtEmail.Text)
msgbox("Company record has been entered.", , "Company Record")
End Sub
Private Sub cmdDisplay_Click(sender As Object, e As EventArgs) _
Handles cmdDisplay.Click
strDetails = ""
strDetails &= customer.GetCompany() & vbCrLf
strDetails &= customer.GetAddress01() & vbCrLf
strDetails &= customer.GetAddress02() & vbCrLf
strDetails &= customer.GetTown() & vbCrLf
strDetails &= customer.GetPostCode() & vbCrLf
strDetails &= customer.GetTel() & vbCrLf
strDetails &= customer.GetFax() & vbCrLf
strDetails &= customer.GetEmail()
txtDetails.Text = strDetails
End Sub
Run the program and enter some customer details, click on the Enter Details button to send the data to the customer object, then click on the Display Details button to retrieve the details from the customer object and display them in the Company Details: box. You should see something like the illustration below.
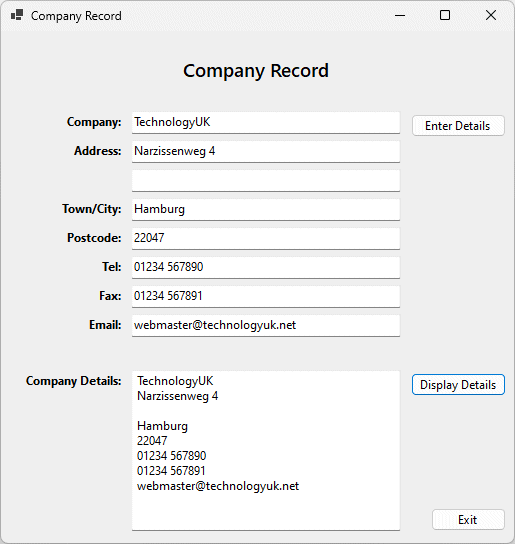
The program output should look something like this